WorldTile System
As for identifiers I want to mention World Tiles. There are a few ways to reference world tiles but two are most useful. Firstly, "x y plane" and second, hashing.
As for X Y Plane, the game is separated into clickable tiles where all things exist. The entire game is tiles, like chess. However there is a z-plane where you can shift up and down on a new tile set. The easiest way to explain it is to go into development worlds and hold CTRL+Scroll up or down or go up a ladder, you will move planes.
Plane 1->plane 2:
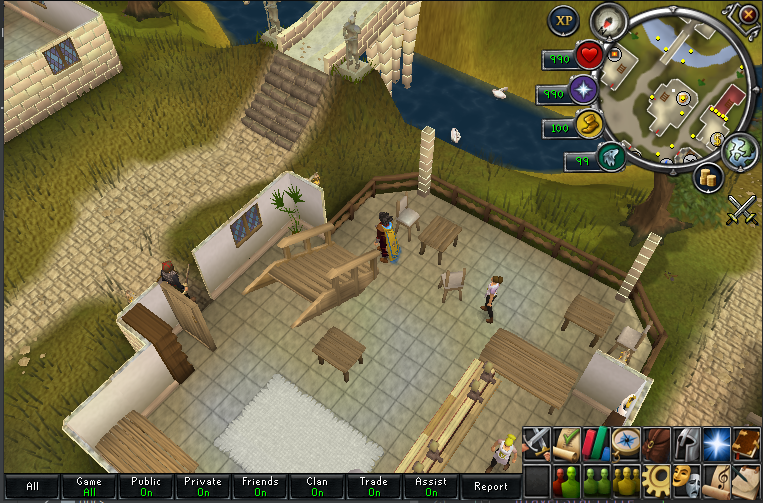
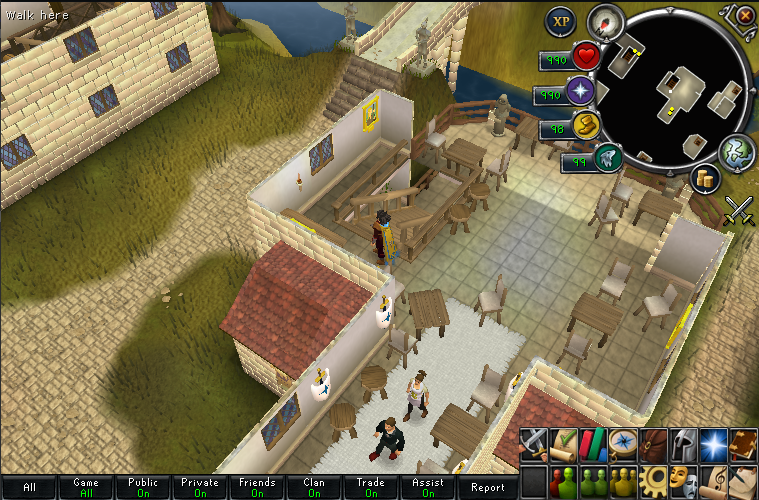
Here is the simplified WorldTile code, See below...
public record WorldTile(short x, short y, byte plane) {
public WorldTile(short x, short y, byte plane) {
this.x = x;
this.y = y;
this.plane = plane;
}
public static WorldTile of(int x, int y, int plane) {
return new WorldTile((short)x, (short)y, (byte)plane);
}
public int getX() { return this.x; }
public int getY() { return this.y; }
public int getPlane() { return this.plane > 3 ? 3 : this.plane; }
public int getTileHash() {
return this.y + (this.x << 14) + (this.plane << 28);
}
public boolean withinDistance(WorldTile tile, int distance) { /*...*/ }
public boolean matches(WorldTile other) {
return this.x == other.x && this.y == other.y && this.plane == other.plane;
}
}
Notice getTileHash() on line 15. It is the same as the programming technique called hashing, where you can create a different number for every use case, this way you can reference the WorldTile in a different way.
As for the code source, hashing is a bit rare but its good to know.
Updated about 2 years ago