Banking
Each player has a Bank data structure saved inside its Player class. Remember that the player is saved on MongoDB along with all the data structures inside it recursively. All that isn't saved is the transient ones. Look below and notice two things
- Item[][] BankTabs is the container or meat of this data structure
- Player exists within the Bank class as well but it is transient.
- We have plugins handling the interfaces in here as well, look below
@PluginEventHandler
public class Bank {
private Item[][] bankTabs;
private short bankPin;
private transient Player player;
private transient int currentTab;
private transient boolean withdrawNotes;
public Bank() {
bankTabs = new Item[1][0];
}
public void setPlayer(Player player) { this.player = player; }
public Item[][] getBankTabs() { return bankTabs; }
public void deleteItem(int itemId, int amount) { /*...*/ }
public boolean containsItem(int itemId, int amount) { /*...*/ }
public void withdrawItem(int bankSlot, int quantity) {
/*...Uses player above to manipulate inventory from Player class...*/
}
public static ButtonClickHandler handleBankButtons = new ButtonClickHandler(763, e -> {
/*...Handles bank interface...*/
});
}
BankTabs 2D array
This is where the items are stored in this Bank class. Each array inside the array is a bank tab. [[Bank tab 1],[Bank Tab 2],[Bank Tab 3]]. Within the bank tab array each index is the slot. That is about all you need to know about that, the rest of the functions just manipulate this 2D array.
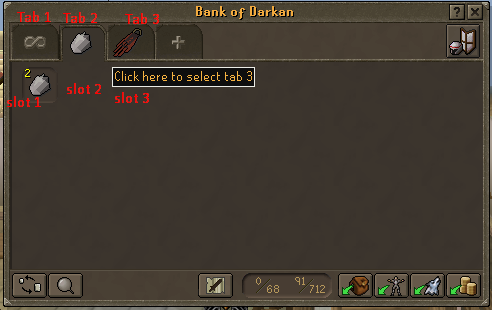
Player is also in the Bank class
The player is stored inside the Bank class to make referencing to the player much much easier and simple. If we had to put the player in every function to manipulate their bank, it would be awfully messy.
When instantiating the Bank class for the first time setPlayer(Player player) is used to assign a player to that bank. If you observe above, you should also notice the player is transient. If the class were not, the player would recursively save when being placed into MongoDB.
Plugins are used to handle bank interfaces
There are ButtonClickHandlers in Inventory.java, Equipment.java, Bank.java and other classes which have an interface, basically taking in the interface number & manipulating its text components and button components.
Updated over 2 years ago